初めてのPHP ⑩。コントローラー、ビュー、Namespaceの設定
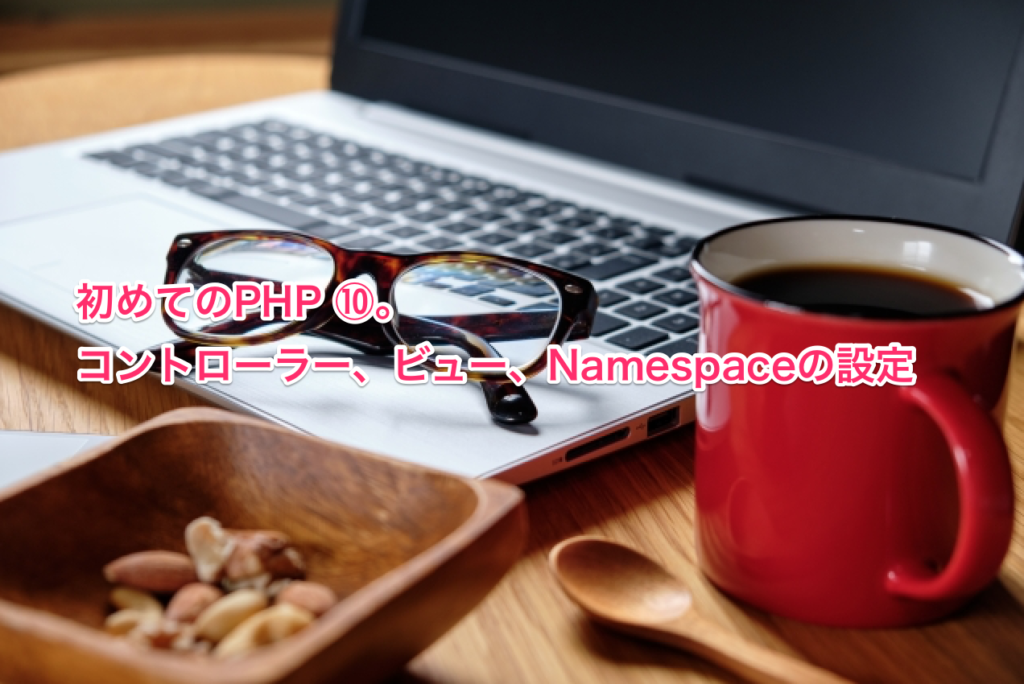
- コントローラー、ビューの設定を分かりやすくしたい
- Namespaceの使い方が知りたい
- PHPを勉強したい
開発が進んでくるとできるだけ綺麗にコードを書かないと見返したときに分かり難くなってしまいます。そこで、コントローラー、ビュー、Namespaceを使ってコードを分かりやすくして行きましょう。
この記事を読むことでコントローラー、ビュー、Namespaceの設定方法が分かります、
初めてのPHP ⑩。コントローラー、ビュー、Namespaceの設定
ここまででコントローラーを設定してきました。
─── PHP-start
├── controllers
├── comment.php
├── delete.php
├── index.php
コントローラーが3つにばらけているのでまとめて行きます。
まずは各コントローラーの内容の確認です。
comment.php
<?php
$error_message = App::get('database')->validate($_POST['comment']);
if(empty($error_message)){
App::get('database')->insert('Comments', [
'comment' => $_POST['comment']
]);
header('Location: /');
}else{
$Comments = App::get('database')->selectAll('Comments');
require 'view/view.php';
}
delete.php
<?php
App::get('database')->delete('Comments', [
'id' => $_GET['id']
]);
header('Location: /');
index.php
<?php
$Comments = App::get('database')->selectAll('Comments');
require 'view/view.php';
CommentController.php
3つをCommentControllerの中に入れて行きます。
comment.phpの内容をsaveメソッド、index.phpの内容をhomeメソッド、delete.phpの内容をdeleteメソッドにします。
class CommentController{
public function home(){
$Comments = App::get('database')->selectAll('Comments');
require 'view/view.php';
}
public function save(){
$error_message = App::get('database')->validate($_POST['comment']);
if(empty($error_message)){
App::get('database')->insert('Comments', [
'comment' => $_POST['comment']
]);
header('Location: /');
}else{
$Comments = App::get('database')->selectAll('Comments');
require 'view/view.php';
}
}
public function delete(){
App::get('database')->delete('Comments', [
'id' => $_GET['id']
]);
header('Location: /');
}
}
routes.phpも編集します。
コントローラー名とメソッド名を@で区切って行きます。
<?php
$router->get('', 'CommentController@home');
$router->get('delete', 'CommentController@delete');
$router->post('comment', 'CommentController@save');
このroutes.phpをindex.phpで呼び出しています。
return Router::load('routes.php')->direct(Request::uri(), Request::method());
routes.phpを編集してのでdirectメソッドも変更してやる必要がありますね。
Routerのdirectメソッドを編集する
<?php
class Router{
public $routes = [
'GET' => [],
'POST' => []
];
public static function load($file){
$router = new static;
require $file;
return $router;
}
public function get($uri, $controller){
$this->routes['GET'][$uri] = $controller;
}
public function post($uri, $controller){
$this->routes['POST'][$uri] = $controller;
}
public function direct($uri, $method){
if(array_key_exists($uri, $this->routes[$method])){
// return $this->routes[$method][$uri];
return $this->callAction(...explode('@', $this->routes[$method][$uri]));
}
throw new Exception('No route');
}
public function callAction($controller, $action){
$controller = new $controller;
if(! method_exists($controller, $action)){
throw new Exception('no respond');
}
return $controller->$action();
}
}
routes.phpで指定したCommentController@homeを@で分解してcallActionメソッドに渡しています。さらに、callActionメソッドではCommentControllerオブジェクトをインスタンス化してhomeやsaveメソッドを呼び出すようにしています。
また、index.phpでrequireとしていたところをreturnにします。homeやsaveメソッド内でrequireやheaderしているのでreturnにします。
// require Router::load('routes.php')->direct(Request::uri(), Request::method());
return Router::load('routes.php')->direct(Request::uri(), Request::method());
Viewの呼び出し
今までrequireを使ってビューを呼び出していましたが、これだと引数を渡す時などに使い難くなります。
そこで、viewという関数を作ってビューを表示させるように設定して行きます。
<?php
class CommentController{
public function home(){
$Comments = App::get('database')->selectAll('Comments');
// require 'view/view.php';
return view('view', compact('Comments')); // 変更
}
public function save(){
$error_message = App::get('database')->validate($_POST['comment']);
if(empty($error_message)){
App::get('database')->insert('Comments', [
'comment' => $_POST['comment']
]);
header('Location: /');
}else{
$Comments = App::get('database')->selectAll('Comments');
// require 'view/view.php';
return view('view'); // 変更
}
}
public function delete(){
App::get('database')->delete('Comments', [
'id' => $_GET['id']
]);
header('Location: /');
}
}
では、viewメソッドを作って行きましょう。
function view($name, $data = []){
extract($data);
return require "view/{$name}.php"
}
extractで第二引数を表示できるようにしています。
Namespaceの設定
開発を続けていくと同じ名前の関数を作ってしまうことがあります。この場合、どちらの関数かわからなくなるのでNamespaceを設定して行きます。
例えば、コントローラーのには下記のようなNamespaceを設定します。
Namespace App\Controller;
このNamespaceの関数を呼び出すためには下記のように設定します。
use App\Controller\CommentController;
まとめ
今回は今まで作ってきたコードの整理を行いました。
こういった設定をしておけば大分開発しやすくなると思います。